How to Create Custom Ansible Wildfly Module – Part 1
Introduction
As promised, I will bring the topic about creating ansible module for Wildfly management. Let’s call it Ansible Wildfly module. Take a note here, this is not about installing Wildfly on the target machine.
Wildfly is one of the most popular Java EE application server, and it is free and open source software. More about Wildfly, please visit their website.
Since I intend to make four custom Ansible modules for Wildfly management, then I will try to split into, unsurprisingly, four parts. In this part, I will cover only how to create and maintain Wildfly Server Group.
Server Group
A server group is set of server instances that will be managed and configured as one.¹
Wildfly itself has two different modes, which are standalone and domain mode. Yet server group only works in domain mode.
There are three ways, as far as I know, for maintaining server group.
- Configuration in
domain.xml
file. This way can be automated via Ansible, but too much pain - Web management console. This is great for user to administer, but almost zero possibility if we want to automate.
- JBoss command-line-interface (CLI) as administration tools. This is a very suitable candidate for Ansible scripting.
Cheat Sheet
This how-to is running on Python 2.7, JDK 8, Wildfly 10.0.0 and using JBoss CLI management guide from this link.
Here is the syntax to execute jboss-cli in shell and we will translate it into python program.
1 2 3 4 5 |
sh $WILDFLY_HOME/bin/jboss-cli.sh \ -c [command line syntax] \ --controller=[host]:[port] \ -u=[user] \ -p=[password] |
Use Case
I want to automate Wildfly Server Group management using Ansible. So here is what the plugin should do:
- Create server group
- Start server group
- Stop server group
- Remove server group
Of course you can use Ansible built-in modules for managing the server group, which are shell and command module. However, my opinion would say the playbook is going to be clumsy, because you have to register output here and there. So, here are the steps!
Step by Step
Step 1 – Workspace Structure
Create file jcli_servergroup.py
under library folder. If you are wondering why I put the file under library folder, please refer to previous post about folder structure. Then import ansible module utils and subprocess module.
1 2 3 4 |
#!/usr/bin/python from ansible.module_utils.basic import * import subprocess |
Note: subprocess module is required for executing shell command
Step 2 – Create Function to Check Server Group Existence
This function will check if server group with specific name has already been created. If we want to execute from the shell, the syntax would be:
1 2 3 4 |
sh jboss-cli.sh -c /server-group=SERVER_GROUP_NAME:query \ --controller=[host]:[port] \ -u=[user] \ -p=[password] |
This syntax will return error code WFLYCTL0216
if the server group with the given name does not exist. Therefore we will use this error code in order to determine its existence.
Next is python part. First, write function isServerGroupAlreadyCreated
then pass the cli command into python subprocess module.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
def isServerGroupAlreadyCreated(data): cmd = data['jboss_home'] + '/bin/jboss-cli.sh' cli = "/server-group=%s:query" % (data['server_group_name']) controller = "--controller=%s:%s" % (data['controller_host'],data['controller_port']) user = "-u=%s" % (data['user']) password = "-p=%s" % (data['password']) p = subprocess.Popen(["sh", cmd, "-c", cli, controller, user, password], stdout=subprocess.PIPE) result , err = p.communicate() created = False remoteExists = False if "WFLYCTL0216" in result: created = False else: created = True if "WFLYPRT0053" in result: remoteExists = False else: remoteExists = True return remoteExists, created |
Note:
- We have six dictionary keys, which are
jboss_home
,server_group_name
,controller_host,
controller_port
,user
andpassword
. These keys will be defined in the main method. - Whenever the result contains
WFLYCTL0216
, it is assumed that the server group with given name already created. - Whenever the result contains
WFLYPRT0053
, it is assumed that the remote host cannot be reached.
Step 3 – Create Server Group
Now it is time to create server group. The syntax in the shell terminal would be:
1 2 3 4 5 6 |
sh jboss-cli.sh -c "/server-group=SERVER_GROUP_NAME:add \ (profile=PROFILE_NAME, \ socket-binding-group=SOCKET_BINDING_GROUP_NAME)" \ --controller=[host]:[port] \ -u=[user] \ -p=[password] |
And the now python part. Create new function and named it server_group_present
and translate previous command into python code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
def server_group_present(data): cmd = data['jboss_home'] + '/bin/jboss-cli.sh' exists, created = isServerGroupAlreadyCreated(data) controller = "--controller=%s:%s" % (data['controller_host'],data['controller_port']) user = "-u=%s" % (data['user']) password = "-p=%s" % (data['password']) isError = False hasChanged = True meta = {} if not exists: mesg = "Could not connect http-remoting://%s:%s" % (data['controller_host'],data['controller_port']) meta = {"status": "Error", "response": mesg} isError = True hasChanged = False else: if not created: cli = "/server-group=%s:add(profile=%s, socket-binding-group=%s)" % (data['server_group_name'],data['server_group_profile'],data['socket_binding_group']) p = subprocess.Popen(["sh", cmd, "-c", cli, controller, user, password], stdout=subprocess.PIPE) result,err = p.communicate() meta = {"status": "OK", "response": result} else: hasChanged = False resp = "Server group %s already created" % (data['server_group_name']) meta = {"status" : "OK", "response" : resp} return isError, hasChanged, meta |
Note:
- We have additional dictionary keys, which are
server_group_profile
andsocket_binding_group
. - The function first checks if the server group with the given name has already created. If it does, then nothing is created and set the
hasChanged
flag into False. Otherwise, it will create a server group then set thehasChanged
into True. - The same function is checking whether the domain controller existence. If not exist then the function will return an error.
Step 4 – Remove Server Group
If you have create functionality, of course it would make sense to have the opposite functionality, which is remove function. And the syntax for removing server group in the shell is:
1 2 3 4 |
sh jboss-cli.sh -c "/server-group=SERVER_GROUP_NAME:remove" \ --controller=[host]:[port] \ -u=[user] \ -p=[password] |
Then map the command into python function, and named it server_group_absent
.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
def server_group_absent(data): cmd = data['jboss_home'] + '/bin/jboss-cli.sh' exists, created = isServerGroupAlreadyCreated(data) controller = "--controller=%s:%s" % (data['controller_host'],data['controller_port']) user = "-u=%s" % (data['user']) password = "-p=%s" % (data['password']) isError = False hasChanged = True meta = {} if not exists: mesg = "Could not connect http-remoting://%s:%s" % (data['controller_host'],data['controller_port']) meta = {"status": "Error", "response": mesg} isError = True hasChanged = False else: if not created: hasChanged = False resp = "Server group %s does not exist" % (data['server_group_name']) meta = {"status" : "OK", "response" : resp} else: cli = "/server-group=%s:remove" % (data['server_group_name']) p = subprocess.Popen(["sh", cmd, "-c", cli, controller, user, password], stdout=subprocess.PIPE) result,err = p.communicate() meta = {"status": "OK", "response": result} return isError, hasChanged, meta |
Notes:
- There is no additional dictionary key for this function.
- The function checks whether the server group with the given name has already created. If it does, then delete the server group and change the
hasChanged
state into True. Otherwise, nothing is executed and change thehasChanged
state into False. - The same function is checking whether the domain controller existence. If not exist then the function will return an error.
Step 5 – Start and Stop Server Group
For starting and stopping server group, the steps are similar with previous step. So, create two python functions, server_group_start
and server_group_stop
.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
def server_group_start(data): cmd = data['jboss_home'] + '/bin/jboss-cli.sh' controller = "--controller=%s:%s" % (data['controller_host'],data['controller_port']) user = "-u=%s" % (data['user']) password = "-p=%s" % (data['password']) exists, created = isServerGroupAlreadyCreated(data) isError = False hasChanged = True meta = {} if not exists: mesg = "Could not connect http-remoting://%s:%s" % (data['controller_host'],data['controller_port']) meta = {"status": "Error", "response": mesg} isError = True hasChanged = False else: if created: cli = "/server-group=%s:start-servers" % (data['server_group_name']) p = subprocess.Popen(["sh", cmd, "-c", cli, controller, user, password], stdout=subprocess.PIPE) result,err = p.communicate() meta = {"status": "OK", "response": result} else: hasChanged = False resp = "Server group %s does not exist" % (data['server_group_name']) meta = {"status" : "OK", "response" : resp} return isError, hasChanged, meta |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
def server_group_stop(data): cmd = data['jboss_home'] + '/bin/jboss-cli.sh' controller = "--controller=%s:%s" % (data['controller_host'],data['controller_port']) user = "-u=%s" % (data['user']) password = "-p=%s" % (data['password']) exists, created = isServerGroupAlreadyCreated(data) isError = False hasChanged = True meta = {} if not exists: mesg = "Could not connect http-remoting://%s:%s" % (data['controller_host'],data['controller_port']) meta = {"status": "Error", "response": mesg} isError = True hasChanged = False else: if created: cli = "/server-group=%s:stop-servers" % (data['server_group_name']) p = subprocess.Popen(["sh", cmd, "-c", cli, controller, user, password], stdout=subprocess.PIPE) result,err = p.communicate() meta = {"status": "OK", "response": result} else: hasChanged = False resp = "Server group %s does not exist" % (data['server_group_name']) meta = {"status" : "OK", "response" : resp} return isError, hasChanged, meta |
Note:
- Both functions have no additional dictionary key
- As same as the other functions, before executing jboss-cli command they check the server availability and has already created or not.
Step 6 – Define Main Function
In the main function, we collect all the dictionary keys that has been used in the previous steps. We have already eight keys. Those keys will be used as an argument specification in AnsibleModule
instance.
In addition, we need to define state for this Ansible Wildfly module. If you look at the use case, we need four states. So here are the states:
- present: server group is created
- absent: server group is removed
- start: start server group
- stop: stop server group
Now we are ready to define the main function:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
def main(): fields = { "jboss_home" : {"required": True, "type": "str"}, "server_group_name": {"required": True, "type": "str"}, "server_group_profile": { "required": False, "default": "default", "type": "str" }, "socket_binding_group": { "required": False, "default": "standard-sockets", "type": "str" }, "controller_host": { "required": False, "default": "localhost", "type": "str" }, "controller_port": { "required": False, "default": 9990, "type": "int" }, "user" : { "required": True, "type": "str" }, "password" : { "required": True, "type": "str" }, "state": { "default": "present", "choices": ['present', 'absent', 'start', 'stop'], "type": 'str' }, } choice_map = { "present": server_group_present, "absent": server_group_absent, "start": server_group_start, "stop": server_group_stop } module = AnsibleModule(argument_spec=fields) is_error, has_changed, result = choice_map.get( module.params['state'])(module.params) if not is_error: module.exit_json(changed=has_changed, meta=result) else: module.fail_json(msg="Error creating server group", meta=result) |
Step 7 – Finalized the jcli_servergroup.py
The last step for jcli_servergroup.py
is to tell python what is the main method.
1 2 |
if __name__ == '__main__': main() |
Step 8 – play.yml
After defining all those functions, we are ready to execute the playbook.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
- hosts: localhost tasks: - name: Server group jcli_servergroup: jboss_home: /opt/wildfly-10.0.0.Final/ server_group_name: group1 state: present user: wildfly password: password register: hasil - debug: var=hasil |
Just make sure you have Wildfly up and running in domain mode. Then run the playbook by typing executing ansible playbook command.
1 |
ansible-playbook play.yml |
After executing that statement, you will get similar outputs:
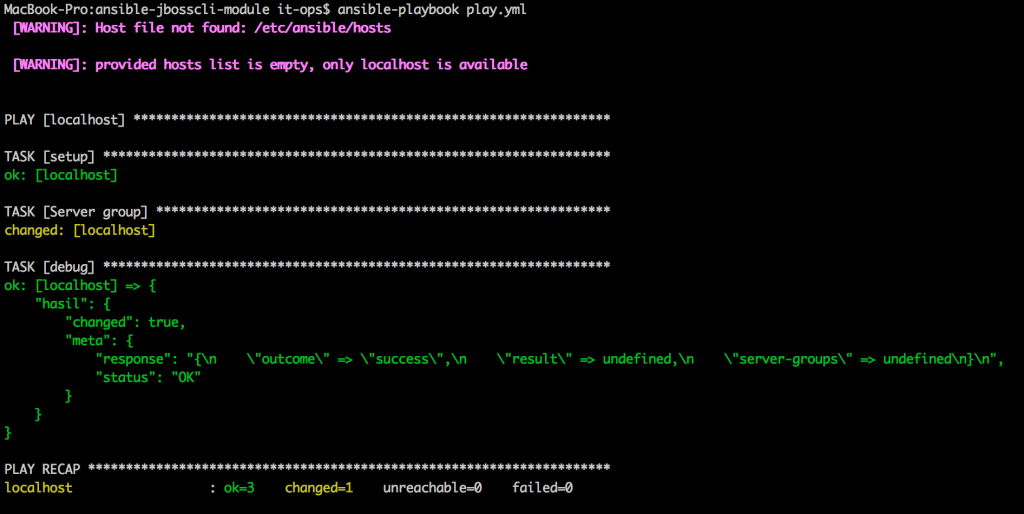
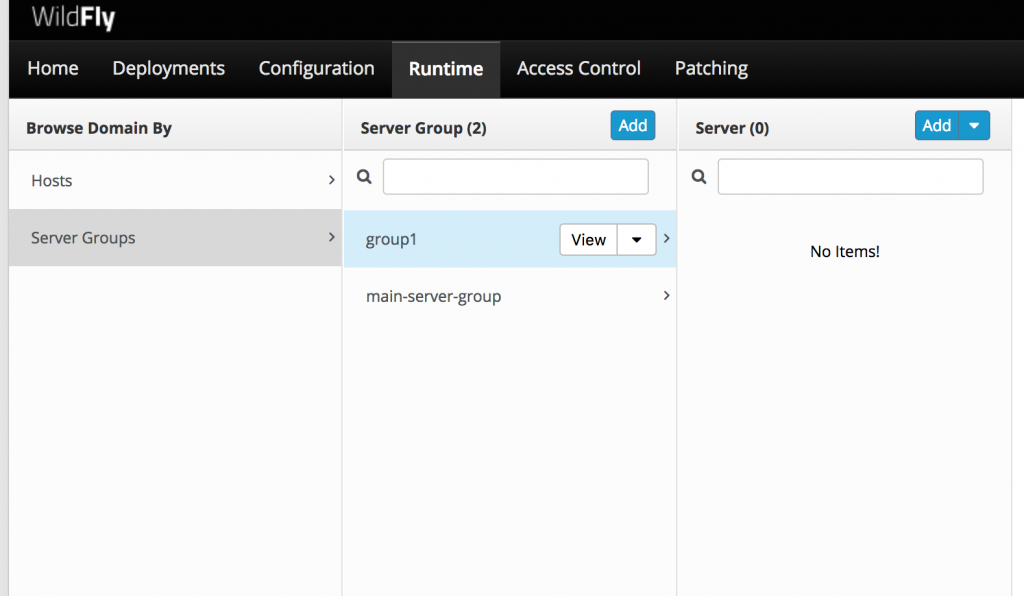
Conclusion
That’s it. Now you can automate Wildfly server group management by using ansible playbook. The next blog, to manage server creation in Wildfly. Hopefully, I can post it sooner than later. Any question or feedback, I’ll see you in comment.
I tried to reproduce what you indicate in the step by step, but it gives me the following error … I am new in python 🙁 ,
sorry
appreciate your help!!!
TASK [Server group] **********************************************************************************************************************************
task path: /root/poc02/play.yml:4
The full traceback is:
Traceback (most recent call last):
File “/usr/lib/python2.7/dist-packages/ansible/executor/task_executor.py”, line 138, in run
res = self._execute()
File “/usr/lib/python2.7/dist-packages/ansible/executor/task_executor.py”, line 558, in _execute
result = self._handler.run(task_vars=variables)
File “/usr/lib/python2.7/dist-packages/ansible/plugins/action/normal.py”, line 46, in run
result = merge_hash(result, self._execute_module(task_vars=task_vars, wrap_async=wrap_async))
File “/usr/lib/python2.7/dist-packages/ansible/plugins/action/__init__.py”, line 694, in _execute_module
(module_style, shebang, module_data, module_path) = self._configure_module(module_name=module_name, module_args=module_args, task_vars=task_vars)
File “/usr/lib/python2.7/dist-packages/ansible/plugins/action/__init__.py”, line 165, in _configure_module
environment=final_environment)
File “/usr/lib/python2.7/dist-packages/ansible/executor/module_common.py”, line 910, in modify_module
environment=environment)
File “/usr/lib/python2.7/dist-packages/ansible/executor/module_common.py”, line 702, in _find_module_utils
recursive_finder(module_name, b_module_data, py_module_names, py_module_cache, zf)
File “/usr/lib/python2.7/dist-packages/ansible/executor/module_common.py”, line 467, in recursive_finder
tree = ast.parse(data)
File “/usr/lib/python2.7/ast.py”, line 37, in parse
return compile(source, filename, mode, PyCF_ONLY_AST)
File “”, line 8
cmd = data[‘jboss_home’] + ‘/bin/jboss-cli.sh’
^
SyntaxError: invalid syntax
fatal: [wildfly01]: FAILED! => {
“msg”: “Unexpected failure during module execution.”,
“stdout”: “”
}
to retry, use: –limit @/root/poc02/play.retry
sudo pip install ansible?
Hi 🙂
Thanks a lot for the good work and explainations 🙂
I’m starting with python with that.
I begin working on it and add some user cases.
For example for server-creation i adds system-properties and jvm heap-sizes features to fill my needs.
I want to check in ‘present’ that definitions are the same than thoses defined in yml file, but work still in progress.
Please let me know if you want I send it to you 🙂
Regards,
Cédric
It would be nice if you publish it!